In our last tutorial, we learned about the NUNit TestFixture attribute. In this tutorial, we learn about the NUnit TestCase attribute and its usage.
The TestCase attribute is used for two purposes.
- It marks a method to test.
- Pass arguments / parameters to the test method.
Test Method
When we assign the TestCase attribute to any method, it lets the NUnit test runner discover this method as a test method so that the NUnit test runner can execute it later.
Below is an example of a NUnit test case.
namespace EmployeeService
{
public class Employee
{
public int Id { get; set; }
public string Name { get; set; }
public int Age { get; set; }
public bool IsSeniorCitizen()
{
if(Age >= 60)
{
return true;
}
return false;
}
}
}
using NUnit.Framework;
namespace EmployeeService.Tests
{
[TestFixture]
public class EmployeeTests
{
[TestCase]
public void When_AgeGreaterAndEqualTo60_Expects_IsSeniorCitizeAsTrue()
{
Employee emp = new Employee();
emp.Age = 60;
bool result = emp.IsSeniorCitizen();
Assert.That(result == true);
}
}
}
NUnit TestCase Arguments and Parameters
TestCase arguments are used when we have to use the same test case with different data.
For example, in the above case, we fixed the age of the employee as 60. For different ages, we have to write different test cases. But by using the TestCase parameters, we can use the same test method for different ages.
[TestCase(60)]
[TestCase(80)]
[TestCase(90)]
public void When_AgeGreaterAndEqualTo60_Expects_IsSeniorCitizeAsTrue(int age)
{
Employee emp = new Employee();
emp.Age = age;
bool result = emp.IsSeniorCitizen();
Assert.That(result == true);
}
In this example, we have used three TestCase attributes on the same method with different parameters. The NUnit framework will create three different test cases using these three parameters.
NUnit TestCase ExpectedResult
In the above example, we have fixed the result to true, which means we can only check the above test case with positive parameters. But by using the ExpectedResult property, we can also specify different results for different parameters. Below is an example:
[TestCase(29, ExpectedResult = false)]
[TestCase(0, ExpectedResult = false)]
[TestCase(60, ExpectedResult = true)]
[TestCase(80, ExpectedResult = true)]
[TestCase(90, ExpectedResult = true)]
public bool When_AgeGreaterAndEqualTo60_Expects_IsSeniorCitizeAsTrue(int age)
{
Employee emp = new Employee();
emp.Age = age;
bool result = emp.IsSeniorCitizen();
return result;
}
In this example, we change the return type of the method to the bool data type and also change the last line of the test case method to the return statement. In the parameters, we specify the expected result as a bool data type matching the return type of the test method.
Author Property
We can specify the author name in the test method who has written the test case. Below is an example:
[TestCase(Author = "Michael")]
public void When_AgeGreaterAndEqualTo60_Expects_IsSeniorCitizeAsTrue()
{
...
}
[TestCase(Author = "George")]
public void When_AgeGreaterAndEqualTo100_Expects_IsSeniorCitizeAsTrue()
{
...
}
For executing tests, right-click on any test method and choose GroupBy -> Traits.
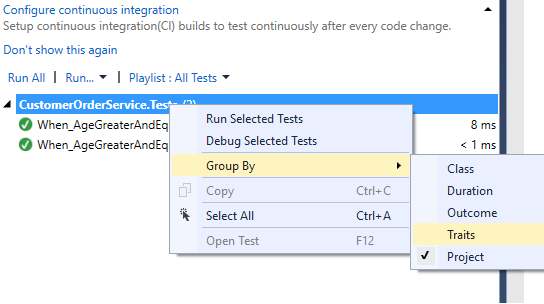
By choosing this option, Test Explorer categorized test methods according to different properties of TestCase. Below is an example of the author property group.
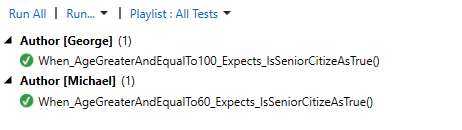
TestName property
The TestName property is used when we have to use a different name than the specified test method name. Below is an example:
[TestCase(TestName = "EmployeeAgeGreaterAndEqualTo60_Expects_IsSCitizenAsTrue")]
public void When_AgeGreaterAndEqualTo60_Expects_IsSeniorCitizeAsTrue()
{
...
}
[TestCase(TestName = "EmployeeAgeGreaterThan100_Expects_IsSCitizenAsTrue")]
public void When_AgeGreaterThan100_Expects_IsSeniorCitizeAsTrue()
{
...
}
Ignore TestCase
Sometimes we need to ignore our test case because the code is not yet complete. So we can use the ignore property to mark the test case as ignored. This still shows the test method in Test Explorer, but Test Explorer will not execute it.
[TestCase(Ignore = "Code is not complete yet.")]
public void When_AgeGreaterAndEqualTo60_Expects_IsSeniorCitizeAsTrue()
{
....
}
In the next tutorial, we learn more about TestCase properties, like how to pass an array to the TestCase method and control the execution order.