1. What is WPF?
Windows Presentation Foundation (WPF) is a successor of Windows Forms. WPF is a UI presentation framework for developing Windows based applications. It uses the DirectX technology for rendering their controls on the screen.
2. What are the features of WPF?
WPF main features are:
- WPF is resolution-independent.
- It uses the hardware acceleration for graphics rendering.
3. What are main WPF assemblies?
There are three WPF assemblies:
- WindowsBase.dll
- PresentationCore.dll
- PresentaitonFoundation.dll
4. Describe the WPF Architecture.
WPF architecture consists of two layers:
- Managed Layer –Â Managed Layer composed of three assemblies:
- WindowsBase
- PresentationCore
- PresentationFramework
- Unmanaged Layer –Â Unmanaged Layer composed of two assemblies:
- MilCore
- WindowsCodec
5. What is the use of WindowsBase Assembly?
WindowsBase assembly contains four important types of WPF.
- DispatcherObject – Base class for all WPF types
- DependencyObject – It’s enables WPF property system services on its derived classes.
- DependencyProperty – It represents a property which can be set through Styles, Data Binding, Animation. It’s inherit values from parent element and provides change notification.
- DispatcherTimer – A special timer class which Tick event method executes on Dispatcher Queue.
6. What is PresentationCore Assembly?
PresentationCore assembly provides Visual class which all the visual controls in WPF are inherit. This assembly directly interacts with MilCore unmanaged assembly for rendering the visual system.
7. What is PresentationFramework Assembly?
PresentationFramework assembly contains all the UI controls like TextBlock, Label, CheckBox, TextBox, ListView, DataGrid.
8. What is MilCore Assembly?
MilCore assembly directly interacts with DirectX for rendering the visual system of WPF. WPF uses DirectX for rendering their display. DirectX is used for efficient hardware and software rendering.
9. What is WindowsCodec Assembly?
WindowsCodec assembly is used for display images in WPF. All imaging display, scaling, transforming is done by WindowsCodec assembly.
10. Describe the WPF classes hierarchy.
Below is the diagram of WPF class hierarchy.
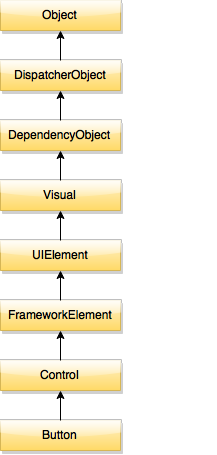
11. What is XAML?
XAML is an XML based language for creating the WPF UI. XAML elements are direct mapping for .NET Objects.
A XAML element name is a .NET class name and XAML element property is the property of that .NET class.
12. Is XAML after compilation convert into Assembly?
Yes. It is stored as BAML (Binary Application Markup Language) into the assembly.
13. What is DependencyProperty?
DependencyProperty is a special type of property introduced in WPF. Only DependencyProperty can participate in Dependency Property System (DPS).
A DependencyProperty compute its value from various inputs at runtime. For example you can set DependencyProperty through Styling, Data binding, animation, and inheritance.
14. Can you write syntax for DependencyProperty?
public static DependencyProperty BackgroundProperty = DependencyProperty.Register("Background", typeof(string), typeof(Customer), new PropertyMetadata("red"), new ValidateValueCallback(ValidateBackgroundProperty));
public string Background
{
get
{
return GetValue(BackgroundProperty) as string;
}
set
{
SetValue(BackgroundProperty, value);
}
}
public static bool ValidateBackgroundProperty(object value)
{
if((string)value == "red" || (string)value == "green")
{
return true;
}
return false;
}
15. How can you give default value to DependencyProperty?
DependencyProperty declaration takes a PropertyMetadata object where we can provide the default value.
16. How can you validate the value of DependencyProperty?
DependencyProperty declaration takes a ValidateValueCallback object. In the object we can define a callback function which execute whenever DependencyProperty changed. Callback function returns bool value if true new value is valid else invalid value.
17. What is Attached Property?
Attach property is special type of DependencyProperty where the property is declared in one class and used in another class.
For example, you can declare AttachedProperty in class MyAttachedProperties and used it with TextBlock, Button, Label objects.
Grid.Row, Grid.Column is a perfect example of Attach Properties.
18. Write down the syntax for Attach Property?
public static readonly DependencyProperty CountProperty = DependencyProperty.RegisterAttached("Count", typeof(int), typeof(MainWindow));
public static int GetCount(DependencyObject obj)
{
return (int)obj.GetValue(CountProperty);
}
public static void SetCount(DependencyObject obj, int value)
{
obj.SetValue(CountProperty, value);
}
19. What is the difference between Attach Property and DependencyProperty?
There is only one difference between Dependency Property and Attach Property.
DependencyProperty is always attached with one class on which it is defined. But Attach Property can attached with any other class.
20. What is ResourceDictionary?
A resourcedictionary is a dictionary class where we can store Styles, DataTemplates and ControlTemplates and any type of resource for later use.
It is just like a CSS file in a HTML page.
ResourceDictionary class inherit from a Dictionary class so we have to store resource as a key value pair.