The array in TypeScript allows you to store a collection of values of a single data type. TypeScript provides a number of functions to do various operations on an array. In this post, we will learn about the TypeScript array and its functions.
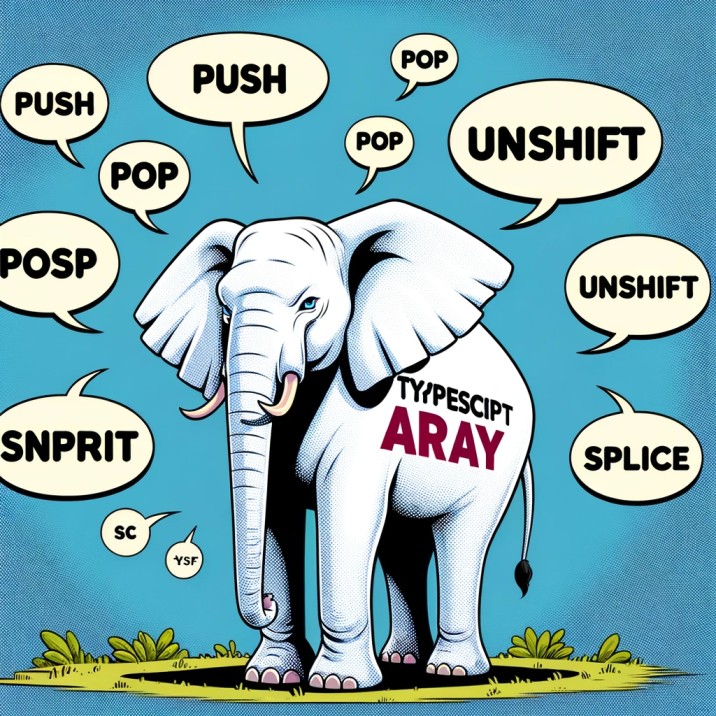
Table of Contents
Syntax
Below is the syntax for declaring an array in TypeScript.
const arr : string[] = ['a','b','c','d'];
In the above example, we have declared an array of type strings. We can store only string data type values in this array. If we try to store another data type, for example, a number, then the TypeScript compiler will throw an error.
We can declare an array of different data types for example: number, boolean, objects etc.
We can access individual elements of an array by an index. The first element of an array always starts with index 0. The rest of the elements will get an index value of 1 plus from the previous element. Below is an example of accessing array elements.
console.log(arr[0]); // "a"
console.log(arr[1]); // "b"
Adding or Removing Elements from an Array
TypeScript provides below functions for adding or removing an elements into array.
- push
- pop
- splice
push() function
The push function is used to add one or more elements into an array.
arr.push('e');
arr.push('f','g','h');
console.log(arr);
Result
======
["a", "b", "c", "d", "e", "f", "g", "h"]
In the first line, we have added only one element into an array and in the second line, we have added three elements in the same push call.
push function add elements always at the end of array.
pop() function
Pop function removes one element from an array. Pop always removes an element from the end of an array. Below is an example.
const arr : string[] = ['a','b','c','d'];
arr.pop();
arr.pop();
console.log(arr);
Result
=======
["a", "b"]
Splice() function
Splice function is a special function which is used to insert and remove elements at particular index in the same call. For example, if we have four elements in an array “a,b,c,d” and we want to add some elements after the b element, then we can use splice function.
Below is the syntax of splice function.
array.splice(index, number_of_elements_to_remove, newelement1, new element2...);
Below are the parameters details:
- index: We can specify the index number from where we need to insert or remove elements.
- number_of_elements_to_remove: We can specify the count of how many elements to remove from the index number.
- newelements…: In the third argument, we can specify n number of elements which we want to add from index number.
Below is an example.
const arr : string[] = ['a','b','c','d'];
arr.splice(2, 2, 'e','f','g');
console.log(arr);
Result
------
["a", "b", "e", "f", "g"]
In above example, we specify index number 2 as first parameter. ‘c’ element comes at index 2. In the second parameter, we specify 2 which means we need to remove two elements starting the index 2 so it will remove elements ‘c’ and ‘d’. After that we specify the new elements which we need to insert.
We can also specify 0 in the second parameter which means we don’t want any element to remove, we only need to insert elements at the specify index.
const arr : string[] = ['a','b','c','d'];
arr.splice(2, 0, 'e','f','g');
console.log(arr);
Result
------
["a", "b", "e", "f", "g", "c", "d"]
In the above example, we specify 0 as second parameter. So TypeScript has not removed any element and insert new elements on index number 2. After putting all the new elements it appends all remaining elements at the end.
Accessing Arrays
We can access the elements in an array by using the two ways below.
- indexers
- for each loop
Indexers
Indexers are used to access single elements from an array. The first element starts with a 0 index. Below is an example of accessing a third argument.
const arr : string[] = ['a','b','c','d'];
console.log(arr[2]);
Result
-------
"c"
For each loop
The for each loop allows you to access all elements of an array in TypeScript.
const arr : string[] = ['a','b','c','d'];
arr.forEach(function(a) {
console.log(a);
});
Result
------
"a"
"b"
"c"
"d"
There is an alternative way to write for each loop using for..of loop. Below is an example.
for (let ele of arr) {
console.log(ele);
}
Check length of an Array
Length Property
To check the total items (length) of an array, we can use the length property of the array. Below is an example.
const arr : string[] = ['a','b','c','d'];
console.log(arr.length);
Result
------
4
Modify Array
For modifying an existing array, we can do the below operations:.
- Insert at specific index
- Replace element value
- Remove element
Insert at specific Index
To insert a new element-specific index, we can use the splice function. Below is an example.
const arr : string[] = ['a','b','c','d','f'];
arr.splice(4, 0, 'e');
console.log(arr);
//Result
["a", "b", "c", "d", "e", "f"]
Replace element Value
To replace an existing value at a specific index, use the indexer. Below is an example.
const arr : string[] = ['a','b','c','d','e','f'];
arr[3] = '123';
console.log(arr);
Result
------
["a", "b", "c", "123", "e", "f"]
In the above example, we have replaced the third element “d” with value “123”.
Remove element
To remove an element, we can again use the splice function.
const arr : string[] = ['a','b','c','d','e','f'];
arr.splice(4, 2);
console.log(arr);
Result
------
["a", "b", "c", "d"]
In the above example, we remove the last elements from an array. We have provided the index number at the splice first parameter and the number of elements to remove at the second parameter.
Search/Filter Array Elements
To search for or filter array elements, TypeScript provides the below functions:.
- IndexOf
- Find
- Filter
IndexOf
The IndexOf function returns the index number of an element. If it has not found that element, then this function returns a -1 value. There are two usages of the IndexOf function.
- To check the existence of a value in an array.
- To get the index number of a value.
Below is an example of checking the existence of an element.
const arr : string[] = ['a','b','c','d','e','f'];
let index = arr.indexOf("d"); //return 3
console.log(index);
index = arr.indexOf("N"); //return -1
console.log(index);
Result
------
3
-1
IndexOf is mainly used with the if statement to check if a value exists in an array of not. Below is an example.
const arr : string[] = ['a','b','c','d','e','f'];
if (arr.indexOf("d") > -1) {
console.log("value exists");
}
Find
The find method is mainly used when we store complex objects in an array and need to find an element based on some condition.
For example, we store objects in an array with two properties: id and name. Now, we want to find an element whose ID property value is 3. We can do this easily with the find function in TypeScript.
interface User {
id: number;
name: string;
}
const users: User[] = [
{ id: 1, name: 'name 1' },
{ id: 2, name: 'name 2' },
{ id: 3, name: 'name 3' },
];
const user = users.find(user => user.id === 3);
console.log(user);
Result
------
{ id: 2, name: 'name 3' }
Filter
The filter method is used when we need to create a new array that contains all the elements of an existing array that satisfy a particular condition.
For example, we need to create a new array that only contains those user objects whose names start with the letter ‘K’.
const users: User[] = [
{ id: 1, name: 'Ramesh' },
{ id: 2, name: 'Suresh' },
{ id: 3, name: 'Kapil' },
{ id: 4, name: 'Kali' }
];
const kUsers = users.filter(w => w.name.startsWith('K'));
console.log(kUsers);
Result
-------
[{
"id": 3,
"name": "Kapil"
}, {
"id": 4,
"name": "Kali"
}]
Sorting in Array
The sort function allows you to sort all elements of an array in TypeScript. By default, the sort function sorts the elements in ascending order.
The sort function sorts the original variable and does not return any value.
Ascending Order
const numbers = [ 4, 5, 6, 3, 2, 1];
numbers.sort();
console.log(numbers);
Result
------
[1, 2, 3, 4, 5, 6]
Descending Order
To sort an array in descending order in TypeScript, we can use the callback function in the sort function. Below is an example.
const numbers = [ 4, 5, 6, 3, 2, 1];
numbers.sort((a,b) => b - a);
console.log(numbers);
Result
------
[6, 5, 4, 3, 2, 1]
Sort Objects By Property
To sort objects by a number property, check out the below example.
const users: User[] = [
{ id: 5, name: 'name 1' },
{ id: 2, name: 'name 2' },
{ id: 7, name: 'name 3' },
];
users.sort((a,b) => a.id - b.id);
console.log(users);
Result
------
[{
"id": 2,
"name": "name 2"
}, {
"id": 5,
"name": "name 1"
}, {
"id": 7,
"name": "name 3"
}]
To sort objects by a string property, use the below example.
const users: User[] = [
{ id: 5, name: 'George' },
{ id: 2, name: 'Michael' },
{ id: 7, name: 'Thach' },
];
users.sort((a,b) => a.name.localeCompare(b.name));
console.log(users);
Result
------
[{
"id": 5,
"name": "George"
}, {
"id": 2,
"name": "Michael"
}, {
"id": 7,
"name": "Thach"
}]
To sort objects by property in descending order, replace the order of objects as shown below.
const users: User[] = [
{ id: 5, name: 'George' },
{ id: 2, name: 'Michael' },
{ id: 7, name: 'Thach' },
];
users.sort((a,b) => b.name.localeCompare(a.name));
console.log(users);
Result
------
[{
"id": 7,
"name": "Thach"
}, {
"id": 2,
"name": "Michael"
}, {
"id": 5,
"name": "George"
}]
Check out all the array functions here.
Summary
The TypeScript array enables storing collections of single data type values, offering various functions for manipulation. These include adding or removing elements through methods like push, pop, and splice, and accessing elements using indexers or loops. The length property returns the array size. Modification functions allow insertion, replacement, or removal at specific indexes. For array element searching or filtering, functions like IndexOf, Find, and Filter are used. Additionally, sorting can be achieved in ascending, descending order, or by object properties, enhancing array management and organization in TypeScript.