In our previous post, we learn how to set up environment for our NUnit Project. In this post, we’ll learn how to write our first NUnit Test Case with Example in .NET / C#.
We have two projects CustomerOrderService project which is a class library and CustomerOrderService.Tests project which is a NUnit test project.
First create two classes Customer and Order and one enum CustomerType in CustomerOrderService project.
namespace CustomerOrderService
{
public enum CustomerType
{
Basic,
Premium,
SpecialCustomer
}
}
namespace CustomerOrderService
{
public class Customer
{
public int CustomerId { get; set; }
public string CustomerName { get; set; }
public CustomerType CustomerType { get; set; }
}
}
namespace CustomerOrderService
{
public class Order
{
public int OrderId { get; set; }
public int ProductId { get; set; }
public int ProductQuantity { get; set; }
public decimal Amount { get; set; }
}
}
CustomerType enum is used for differentiate customers. Some customers are Basic customers and some are Premium or Special Customer.
In the next class CustomerOrderService, we will write our Business Logic to give some discount to Premium or Special Customer types. In the NUnit project, we will write unit test cases for validating the discount logic.
namespace CustomerOrderService
{
public class CustomerOrderService
{
public void ApplyDiscount(Customer customer, Order order)
{
if (customer.CustomerType == CustomerType.Premium)
{
order.Amount = order.Amount - ((order.Amount * 10) / 100);
}
else if(customer.CustomerType == CustomerType.SpecialCustomer)
{
order.Amount = order.Amount - ((order.Amount * 20) / 100);
}
}
}
}
In the above method, we are giving 10% discount to Premium customers and 20% discount to Special Customers. We are not giving any discount to Basic customers.
Now, come under CustomerOrderService.Tests project. Create a new class “CustomerOrderServiceTests”. Add namespace “using NUnit.Framework” in the namespaces section.
Add [TestFixture] attribute to CustomerOrderTests class. [TestFixture] attribute marks the class that this class contains test methods. Only classes with [TestFixture] attribute can add test case methods.
using NUnit.Framework;
namespace CustomerOrderService.Tests
{
[TestFixture]
public class CustomerOrderServiceTests
{
}
}
Add a new method name “When_PremiumCustomer_Expect_10PercentDiscount” and add [TestCase] attribute.
using NUnit.Framework;
namespace CustomerOrderService.Tests
{
[TestFixture]
public class CustomerOrderServiceTests
{
[TestCase]
public void When_PremiumCustomer_Expect_10PercentDiscount()
{
}
}
}
All test cases method are public and void return because in the test cases we should not return any value.
We should write NUnit test method name in special naming convention. Below is the naming convention I am using in the above test case name.
When_StateUnderTest_Expect_ExpectedBehavior
First part starts with fixed ‘When’. Second part specify the state which we want to test. Third part is also fixed ‘Expect’ and forth part specify the expected behavior of method under test.
Now, we write our first NUnit test case example method code. A test case body is divided into three sections “AAA”.
“AAA” denotes the Arrange, Act, and Assert.
Arrange: In Arrange section, we will initialize everything which we are required to run the test case. It includes any dependencies and data needed.
Act: In Act section, we called the business logic method which behavior we want to test.
Assert: Specify the criteria for passing the test case. If these criteria passed, that means test case is passed else failed.
First NUnit Test Case Example
[TestCase]
public void When_PremiumCustomer_Expect_10PercentDiscount()
{
//Arrange
Customer premiumCustomer = new Customer
{
CustomerId = 1,
CustomerName = "George",
CustomerType = CustomerType.Premium
};
Order order = new Order
{
OrderId = 1,
ProductId = 212,
ProductQuantity = 1,
Amount = 150
};
CustomerOrderService customerOrderService = new CustomerOrderService();
//Act
customerOrderService.ApplyDiscount(premiumCustomer, order);
//Assert
Assert.AreEqual(order.Amount, 135);
}
In Arrange section, we initialize two variables premiumCustomer and order. In the next line, we create an instance of our CustomerOrderService class.
In Act section, we called actual method of CustomerOrderService class with variables initialized in Arrange section.
In Assert section, we check our expected response. Is amount equals to 135 or not?
Assert Class
Assert is NUnit framework class which has static methods to verify the expected behavior. In the above example, AreEqual method verifies that two values are equal or not.
We’ll learn more about Assert class in our next posts.
If the Assert condition is passed then the NUnit test case is passed else failed.
Now we will run our first NUnit test case.
Steps:
- Build the full Solution
- Click on Test Menu -> Windows -> Test Explorer
- Click on Run All link.
‘Run All’ link search entire solution for methods which has [TestCase] attribute and run all those methods.
After Run All, if our test case method name color change to Green, that means our test case is passed, and if it turns into red color that means our test is failed.
Below is our test result.
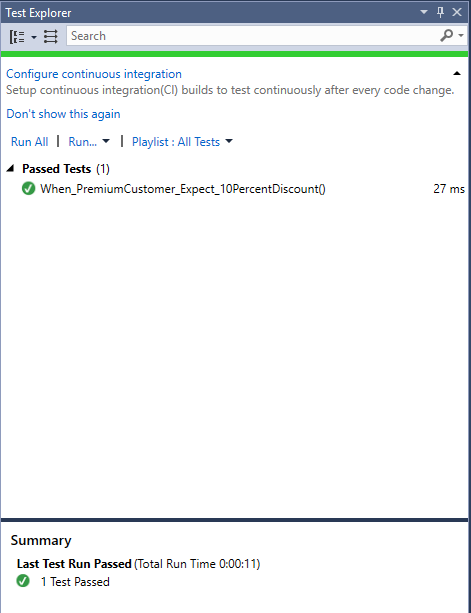
Now if I change the expected result to 130 and again build the project and click on “Run All” link. Then our test case fails.
[TestCase]
public void When_PremiumCustomer_Expect_10PercentDiscount()
{
//Arrange
Customer premiumCustomer = new Customer
{
CustomerId = 1,
CustomerName = "George",
CustomerType = CustomerType.Premium
};
Order order = new Order
{
OrderId = 1,
ProductId = 212,
ProductQuantity = 1,
Amount = 150
};
CustomerOrderService customerOrderService = new CustomerOrderService();
//Act
customerOrderService.ApplyDiscount(premiumCustomer, order);
//Assert
Assert.AreEqual(order.Amount, 130);
}
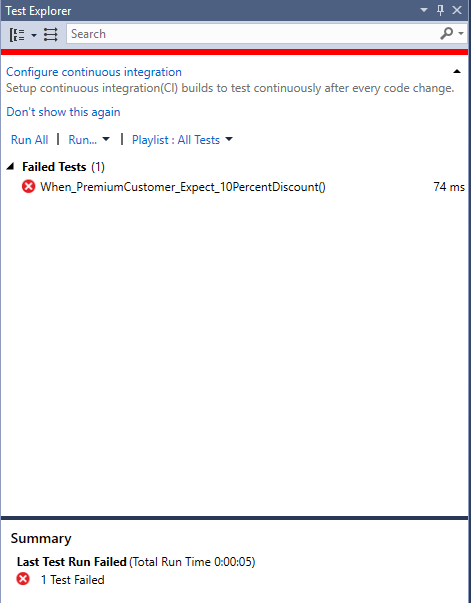
We have just created our first NUnit Test Case example in .NET / C#. We’ll learn more about NUnit Attribute in our next posts.
Kindly put your comments about this post or any questions you want to ask from me.