TabControl provides a ItemsSource property to bind list of items. ItemsSource takes any collection object which implements the IEnumerable interface.
We bind TabControl with the List<T> or ObservableCollection<T> list classes.
Below is the example TabControl binding with list of MyTabItem class.
TabControl Binding Example
Create a new MyTabItem class which have two properties.
- Header
- Content
We’ll set Header property to Header of TabItem and content property to Content of TabItem.
public class MyTabItem
{
public string Header { get; set; }
public string Content { get; set; }
}
Below is the XAML for TabControl.
<Window x:Class="WpfApplication1.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="WPF Tab Control Binding Example" Height="350" Width="525">
<Grid>
<TabControl x:Name="TabControl1" Margin="10" >
<TabControl.ItemContainerStyle>
<Style TargetType="TabItem">
<Setter Property="Header" Value="{Binding Header}" />
<Setter Property="Content" Value="{Binding Content}" />
</Style>
</TabControl.ItemContainerStyle>
</TabControl>
</Grid>
</Window>
In the above TabControl, I have set TabControl.ItemContainerStyle. ItemContainerStyle style is applied to the container element generated for each item.
I have binded Header property of MyTabItem class to the Header property of TabControl and bind Content property of MyTabItem class to the Content property of TabControl.
In the code-behind file, we’ll create a list of MyTabItem classes and set that list to the ItemsSource property of TabControl.
using System.Collections.Generic;
using System.Windows;
namespace WpfApplication1
{
/// <summary>
/// Interaction logic for MainWindow.xaml
/// </summary>
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
List<MyTabItem> tabItems = new List<MyTabItem>();
tabItems.Add(new MyTabItem { Header = "Header 1", Content = "Content 1" });
tabItems.Add(new MyTabItem { Header = "Header 2", Content = "Content 2" });
TabControl1.ItemsSource = tabItems;
}
}
public class MyTabItem
{
public string Header { get; set; }
public string Content { get; set; }
}
}
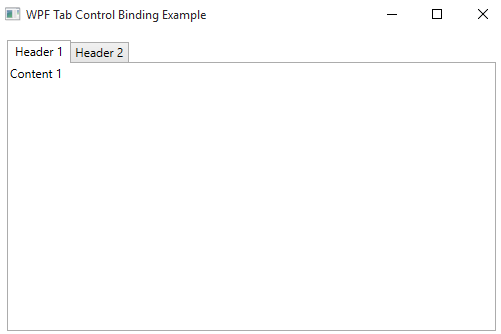
###ADSPACE3###