There are two steps in configuring the NUnit project environment:
- Configure Project with NUnit assemblies
- Setup TestRunners which show the results of NUnit test cases
Configure Project with NUnit assemblies
We always create separate projects when creating projects for NUnit. According to naming conventions, the test project name should be [Project Under Test].[Tests]. For example, if we are testing the project name “CustomerOrderService,” then the test project name should be “CustomerOrderService.Tests.”.
- Visual Studio -> New Project -> Class Library -> Name: CustomerOrderService
- Right-click on solution: Add New Project, Class Library, Name: CustomerOrderService.Tests
Now we have two projects in our solution. In the CustomerOrderService project, we write code for business logic, and in the second project, CustomerOrderService,. Tests: We write test cases for the CustomerOrderService project.
Our next step is to add Nunit assemblies.
- Right-click on CustomerOrderService.Tests and choose “Manage NuGet Packages.”.
- In the NuGet search box, choose the Browse tab and type Nunit in the search textbox.
- Choose NUnit and click on the Install button.
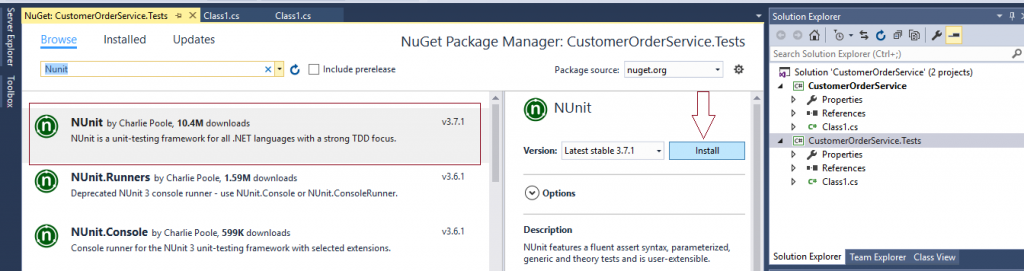
- NUnit assembly (nunit.framework) is added to our test project.
- Add a reference to our CustomerOrderService class library to the test project.
- Choose add reference in test project -> Project -> Solution tab -> Mark the checkbox before the CustomerOrderService button. Click on the OK button.
Now our test project is configured with Nunit assemblies. Our next step is to add TestRunners to our solution.
Setup TestRunners
For setup TestRunners, we need to add Nunit Test Adapter from NuGet packages. Follow the below steps:
- Right-click on CustomerOrderService.Tests and choose ‘Manage NuGet Packages’
- Choose NUnit3TestAdapter and click on the Install button.
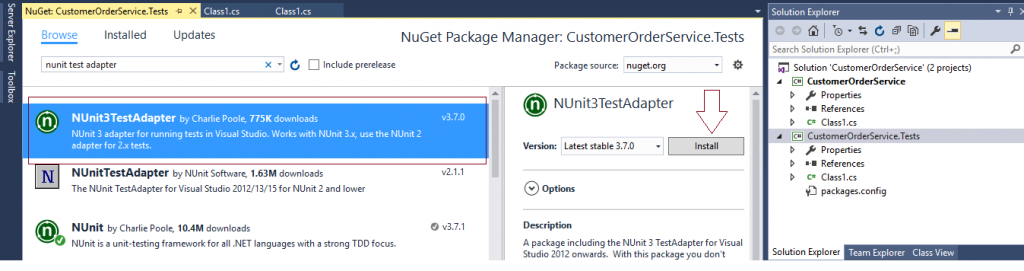
Now we have to write a sample test case to check whether everything is set up successfully or not. Write a sample test case in class 1 of CustomerOrderService.Tests.
using NUnit.Framework;
namespace CustomerOrderService.Tests
{
[TestFixture]
public class Class1
{
[Test]
public void Test1()
{
Assert.That(1 == 1);
}
}
}
Include namespace NUnit.Framework in the namespaces section. Write the above code exactly in class. We’ll learn more about the TestFixture attribute, the Test attribute, and the Assert class in our next posts. Now build the solution.
Choose visual studio Test Menu -> Windows -> Test Explorer.
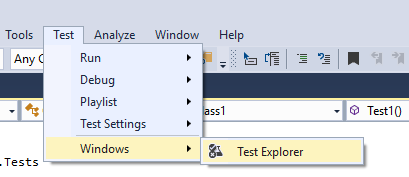
Text Explorer shows the Test function in the Not Run Tests section. Choose the Run All button to execute test cases.
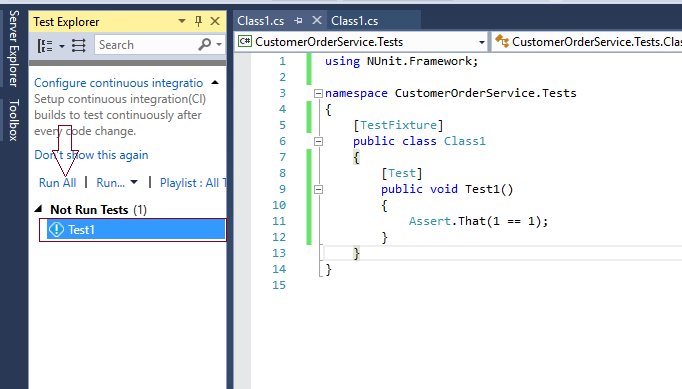
In below of Test Explorer, it will show the result of Test1 test result. In the below screenshot, it is showing the result ‘Test Passed—Test1’.
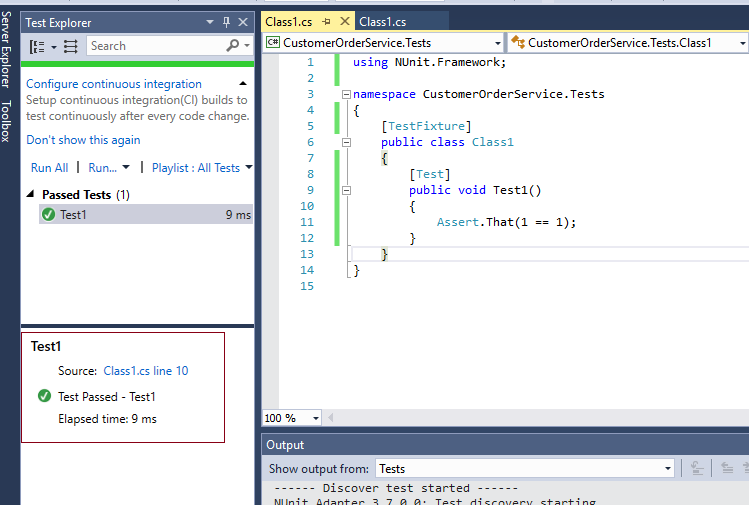
Now our test project and TestRunner are configured properly. In the next few posts, we’ll learn more about Nunit attributes and classes.Â